In this 2nd edition of .NET Fiddle Newsletter, we will tell you the main reason for using Async Await, the easiest way to read/write CSV files, and the solution to the Tower of Hanoi puzzle.
This newsletter was brought to you by “Conan the Barbarian” (1982)
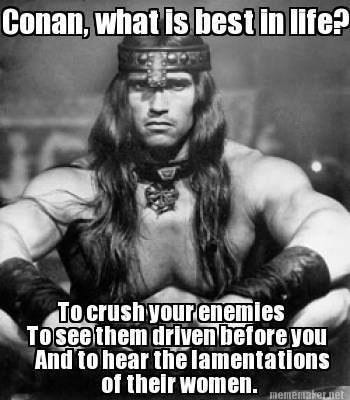
If you are one'em Millenials, you may not have seen this Arnold’s classic. If not, please do me a favor, go ahead and drop everything (unless you are holding a baby), and WATCH IT NOW!! (in Arnold’s voice).
Also "Lamentations" means "the passionate expression of grief or sorrow". You are welcome.
The mystery of Async Await
If you are asked why you need Async Await during an interview and you reply that it is just a simplified way to do Parallel tasks, you will probably get this look from your interviewer:
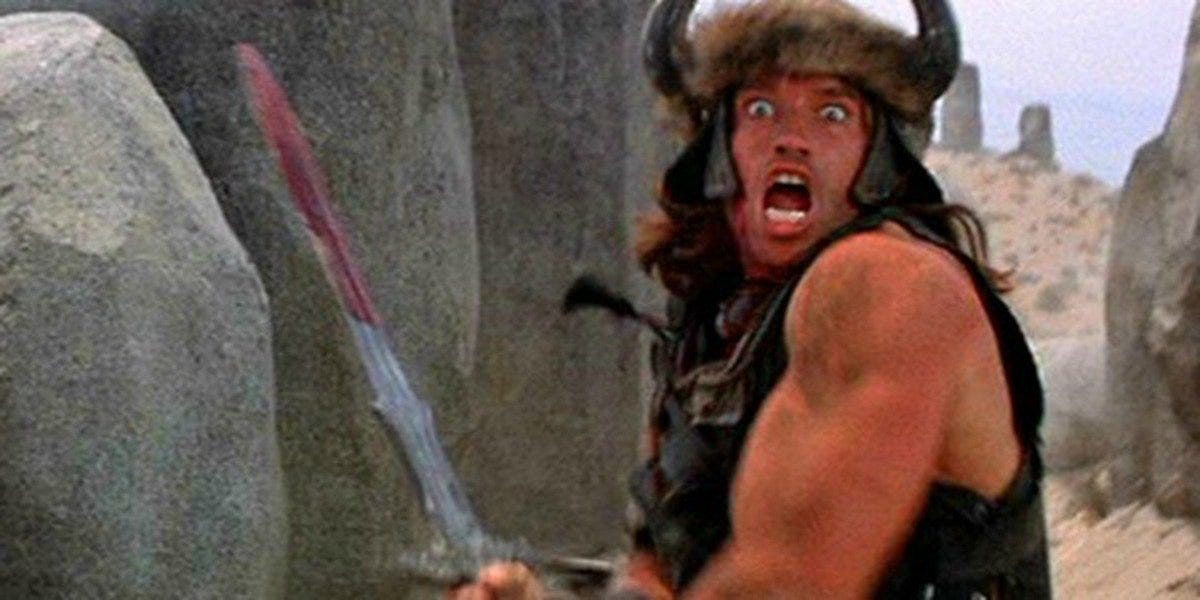
Check out this fiddle to see how Sync programming compares to Async and to Parallel:
https://dotnetfiddle.net/Us071J
Microsoft has pretty good documentation describing asynchronous programming using the “breakfast” metaphor. When you are making breakfast you have to do a bunch of tasks:
Make coffee
Cook Eggs
Toast bread
In Sync programming you would complete each task before starting the next, so you would turn on the coffee maker, but wait till it is boiling before switching to another task. In Async programming, you would turn on the coffee maker, but instead of waiting for it to finish, you would go ahead and start on cooking eggs or toasting bread. In parallel programming, you would need to ask a friend or two, so that all of you could start on one task each and then finish it fully before moving to the next task.
You can read about Asynchronous programming in more detail here:
https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/concepts/async
Re-invent the wheel or use CSVHelper?
If I got a quarter every time I wrote code for writing/reading CSV files, I would have at least a dollar and some change. After 5th time, it started feeling a bit like a “Wheel of Pain”.
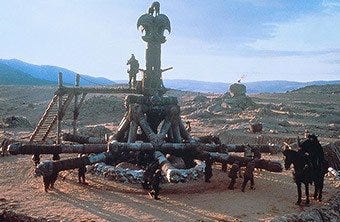
This is The Wheel of Pain, by the way. Once again from “Conan the Barbarian”, and once again, millennials you are missing out.
Instead of visiting the “Wheel of Pain” there is a much better way.
Here is a fiddle that utilizes the CSVHelper NuGet package that makes dealing with CSV files a joy.
https://dotnetfiddle.net/lLMg0W
Solution for Tower of Hanoi Puzzle
In the last newsletter, we presented a fun classic challenge called “Tower of Hanoi”.
You start here:
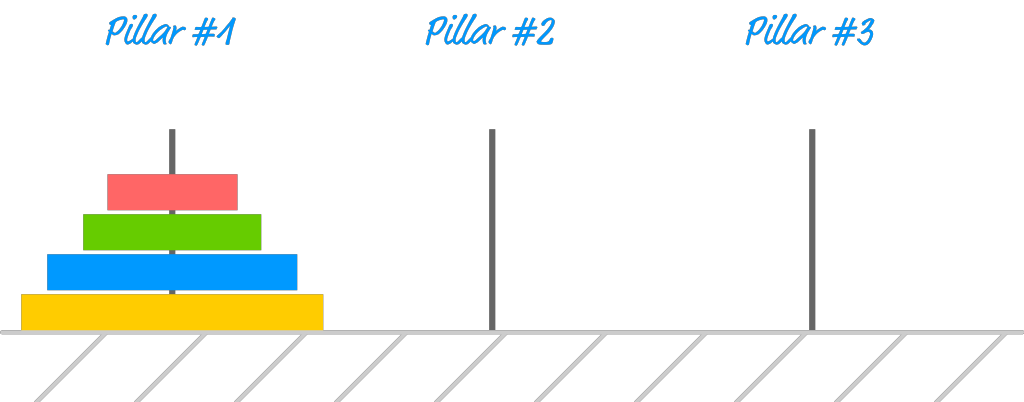
And you need to end up here:
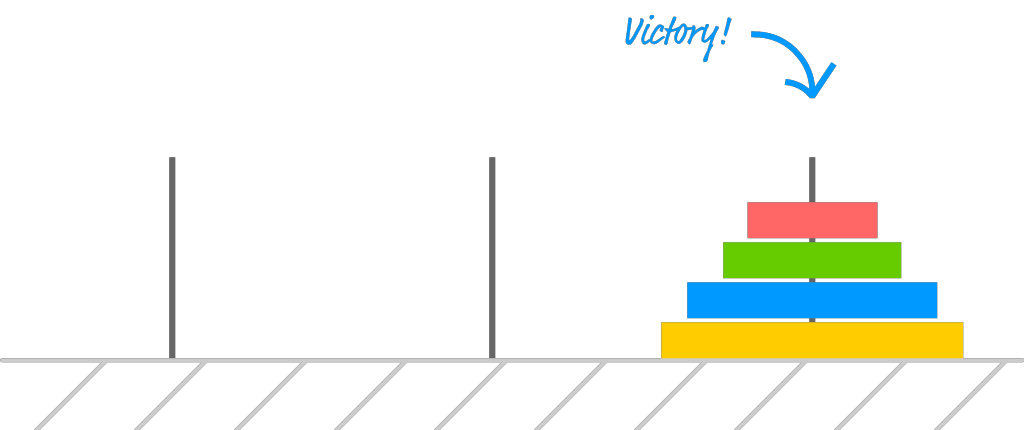
You can move only one disk at a time and you can only place the smaller disk on top of the larger. The solution should work for 3, 4, or more disks.
Here is the fiddle with the solution to this puzzle. It is one of the classic ways to solve this problem by using recursion:
https://dotnetfiddle.net/EHLYsO
You can find alternative solutions such as non-recursive and binary on Wikipedia page: https://en.wikipedia.org/wiki/Tower_of_Hanoi
Sponsored by Panda Invest - Earn 20X more then regular savings account
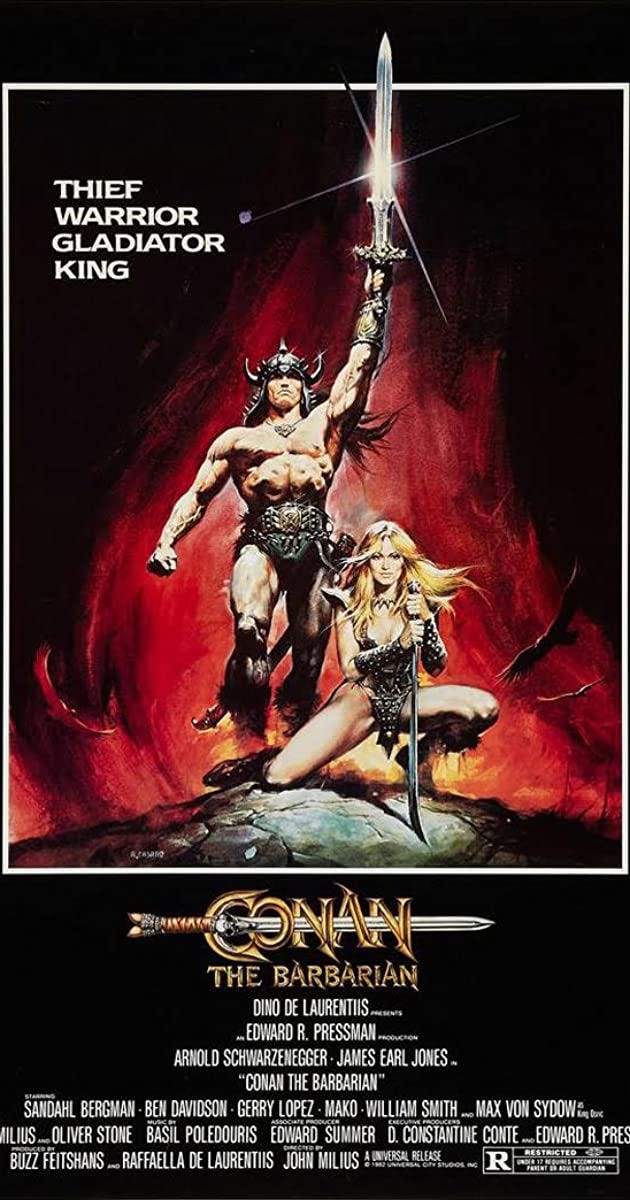
This movie also has one of the best soundtracks of any movie ever.
If you would like to unsubscribe and you don’t want to learn the secrets to great wealth then click on the unsubscribe link below.
If you would like to contribute a cool fiddle or suggest a new topic for a future newsletter, please tweet it to @dotnetfiddle
Try to Add this to the code
public static void MakeBreakTask()
{
Console.WriteLine("\n============================");
Console.WriteLine("\nMakeBreakTasknc");
Console.WriteLine("\nNOTE: tasks maybe using different threads provided by ThreadPool, but unlike parallel programming, it is only one thread at a time\n");
StartTimer();
WriteLog("MakeBreakTask Start");
var boilCoffeeTask = Task.Run( BoilCoffee);
var toastBreadTask = Task.Run(ToastBread);
var fryEggsTask = Task.Run(FryEggs);
Task.WaitAll(new[]{ boilCoffeeTask,toastBreadTask,fryEggsTask});
WriteLog("MakeBreakTask End");
Console.WriteLine($"\nTotal time: {_stopwatch.Elapsed.TotalMilliseconds}ms\n");
}